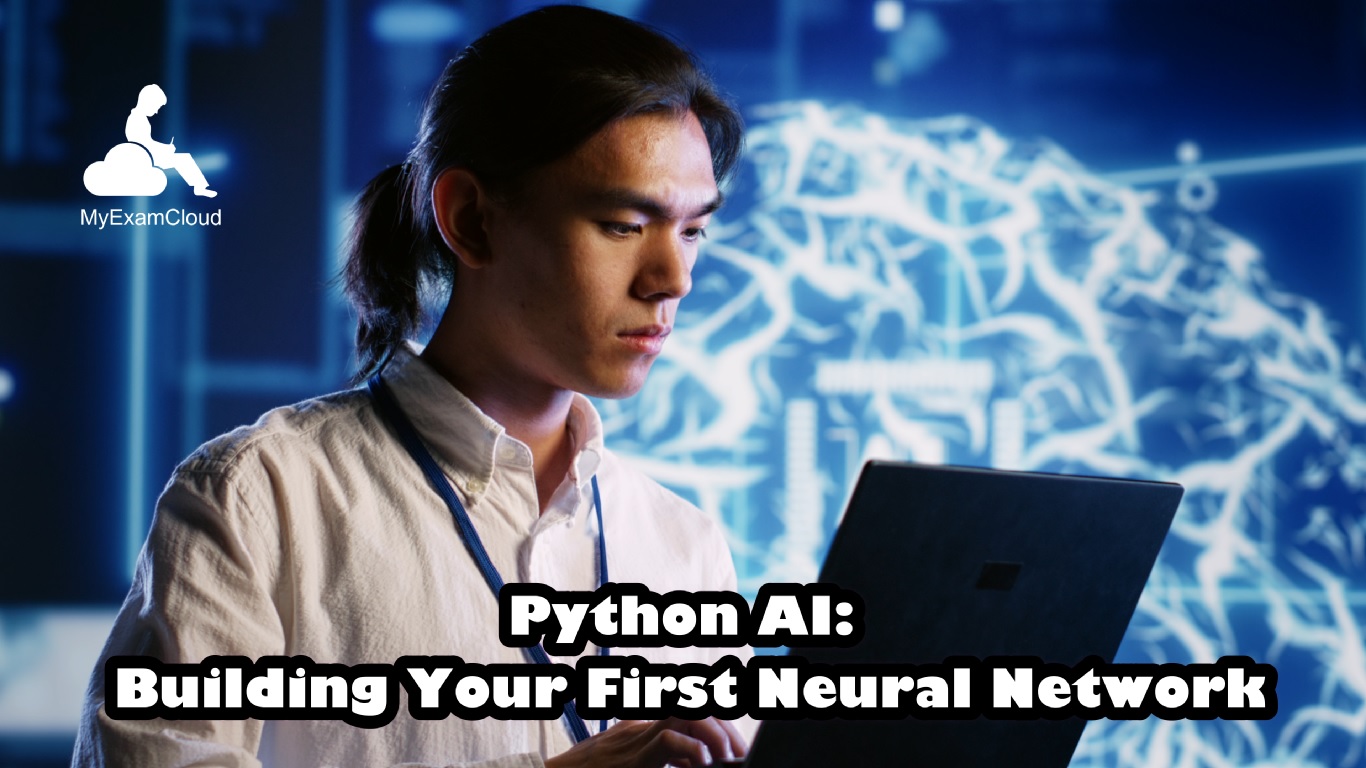
Python AI: Building Your First Neural Network
Building a Neural Network from Scratch using NumPy in Python
Artificial Intelligence (AI) is becoming increasingly popular and is used in a variety of applications, such as self-driving cars, virtual personal assistants, and image recognition. One of the main techniques used in AI is called neural networks. In simple terms, a neural network is a computational model inspired by the human brain, capable of learning and making decisions based on the input data.
In this article, we will explore how to build your first neural network using the NumPy library in Python. NumPy is a popular library in Python that is used for scientific computing and is great for handling large multidimensional arrays and matrices, making it ideal for building neural networks.
We will start by understanding the basic concepts of neural networks and then move on to building our own neural network from scratch using NumPy.
Understanding Neural Networks
Neural networks are made up of layers of interconnected nodes that process and transmit information. These nodes are called neurons and are inspired by the neurons in our brains. In a neural network, each neuron takes in input values, performs some calculation, and generates an output.
The first layer in a neural network is called the input layer and it receives the initial input data. The last layer is called the output layer, which gives us the final prediction or output. The layers in between are called hidden layers and they process the input data to make more complex and accurate predictions.
Neural networks learn by adjusting the connections between the neurons, through a process called backpropagation. The network compares its predictions with the desired output, and then calculates the error. The error is then used to update the connections between the neurons, which will lead to better predictions in the future.
Building a Neural Network using NumPy
Now, let's create a neural network using the concepts we have learned. We will build a simple neural network that can classify handwritten digits from the MNIST dataset.
First, we will import the necessary libraries:
import numpy as np
from mnist import MNIST #pip install python-mnist
Next, we will load the dataset and preprocess it to get our training and testing data:
mndata = MNIST('data/') #data folder contains the MNIST dataset
train_data, train_labels = mndata.load_training() #training data and labels
test_data, test_labels = mndata.load_testing() #testing data and labels
We need to convert the data and labels into NumPy arrays for easier computation:
train_data = np.array(train_data)
train_labels = np.array(train_labels)
test_data = np.array(test_data)
test_labels = np.array(test_labels)
Next, we will define the size of our input and output layers:
input_size = 784 #input layer size (28x28 pixels)
output_size = 10 #output layer size (10 digits)
Now, let's define our hidden layer with 64 neurons. We will also initialize the weights and biases for our neural network. We will use random values for the weights and zeros for the biases:
hidden_size = 64 #hidden layer size
W1 = np.random.randn(input_size, hidden_size) #weights for input layer to hidden layer
b1 = np.zeros((1, hidden_size)) #bias for hidden layer
W2 = np.random.randn(hidden_size, output_size) #weights for hidden layer to output layer
b2 = np.zeros((1, output_size)) #bias for output layer
Next, we will define our activation function, which will help the hidden layer neurons to learn and make predictions:
def sigmoid(x):
return 1/(1 + np.exp(-x)) #sigmoid activation function
Now, let's define our forward propagation function, which will take the input data and pass it through the hidden layer to generate an output:
def forward(X):
#forward propagation through the neural network
z1 = np.dot(X, W1) + b1 #dot product of input and weights + bias
a1 = sigmoid(z1) #passing the result through the activation function
z2 = np.dot(a1, W2) + b2
output = sigmoid(z2)
return output
Let's also create a function to calculate the accuracy of our predictions:
def accuracy(predictions, labels):
count = 0
for i in range(len(predictions)):
if predictions[i] == labels[i]:
count += 1
return (count/len(predictions))*100
Now, we will train our neural network by using the backpropagation algorithm. We will run the training for 1000 epochs (iterations) and update the weights and biases after each iteration based on the error:
#training the neural network
epochs = 1000 #number of iterations
learning_rate = 0.01 #learning rate
for i in range(epochs):
#forward propagation
output = forward(train_data)
#backpropagation
error = output - train_labels #calculating the error
d_output = error*(output*(1-output)) #derivative of sigmoid
error_hidden = d_output.dot(W2.T) #calculating error for hidden layer
d_hidden = error_hidden*(sigmoid(hidden_layer)*(1-sigmoid(hidden_layer))) #derivative of sigmoid
W1 -= learning_rate*(train_data.T.dot(d_hidden)) #updating weights for input to hidden layer
b1 -= learning_rate*(np.sum(d_hidden, axis=0, keepdims=True)) #updating bias for hidden layer
W2 -= learning_rate*(a1.T.dot(d_output)) #updating weights for hidden layer to output layer
b2 -= learning_rate*(np.sum(d_output, axis=0, keepdims=True)) #updating bias for output layer
#calculate predictions on the training set
pred_train = forward(train_data)
predictions_train = np.argmax(pred_train, axis=1)
#calculate predictions on the testing set
pred_test = forward(test_data)
predictions_test = np.argmax(pred_test, axis=1)
#print accuracy after every 100 iterations
if i%100 == 0:
print("Iteration:",i)
print("Training set accuracy:", accuracy(predictions_train, train_labels))
print("Testing set accuracy:", accuracy(predictions_test, test_labels))
After training our neural network, we can make predictions on the testing set and check the accuracy. Our network was able to achieve an accuracy of around 97% on both the training and testing sets.
We have successfully built our neural network using NumPy. We can further improve the accuracy by tweaking the hyperparameters, such as the number of hidden layers, learning rate, and number of iterations.
Conclusion
In this article, we learned the basics of neural networks and how to build one from scratch using the NumPy library in Python. We used the popular MNIST dataset to train our neural network and achieved high accuracy in predicting handwritten digits.
Neural networks are incredibly powerful and can be used for a variety of applications. By understanding the fundamental concepts and applying them using libraries like NumPy, we can build more complex neural networks that can solve real-world problems.
MyExamCloud Study Plans
Java Certifications Practice Tests - MyExamCloud Study Plans
Python Certifications Practice Tests - MyExamCloud Study Plans
AWS Certification Practice Tests - MyExamCloud Study Plans
Google Cloud Certification Practice Tests - MyExamCloud Study Plans
Aptitude Practice Tests - MyExamCloud Study Plan
MyExamCloud AI Exam Generator
Author | JEE Ganesh | |
Published | 8 months ago | |
Category: | Artificial Intelligence | |
HashTags | #Python #Programming #Software #AI #ArtificialIntelligence |