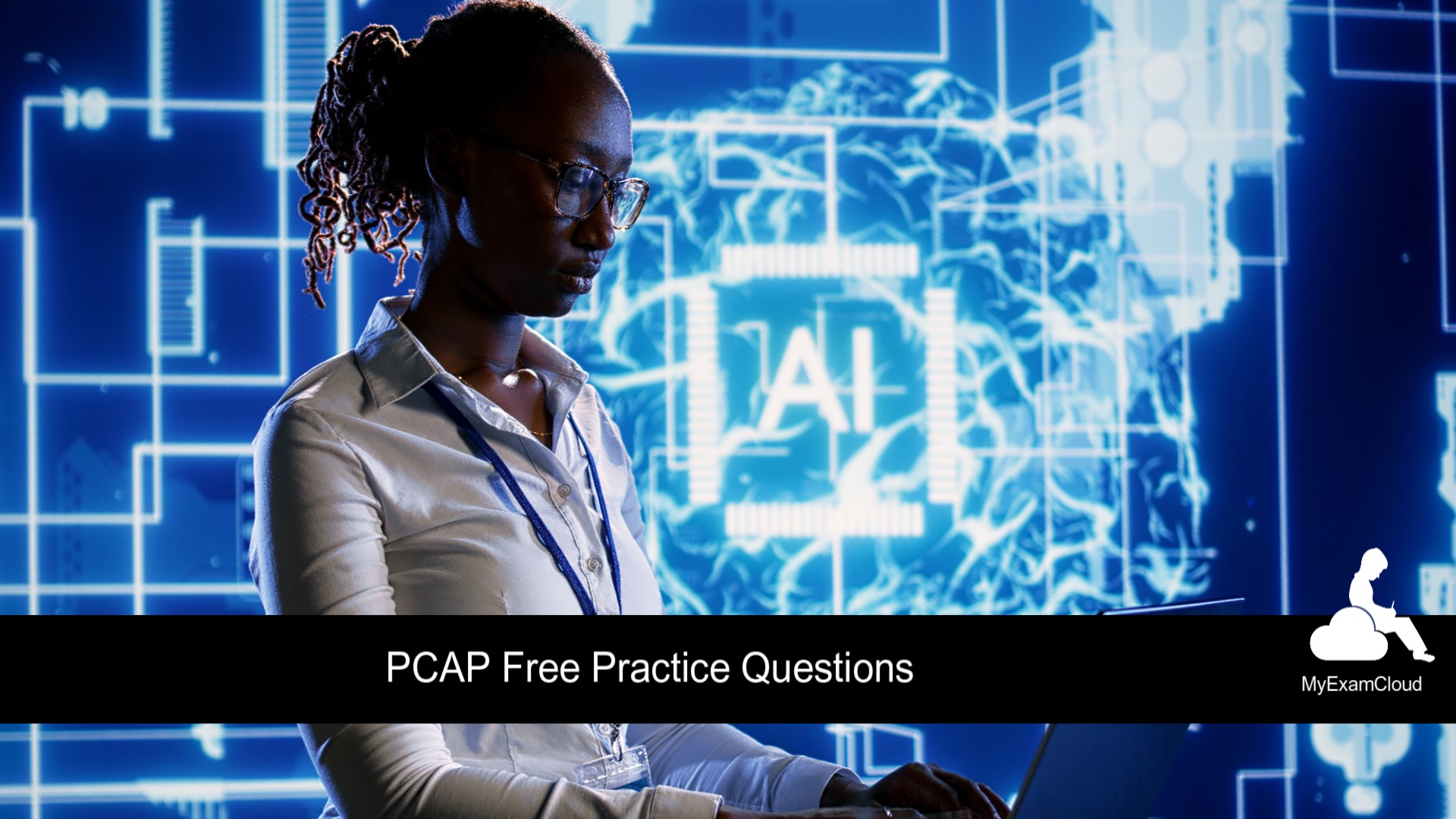
PCAP Free Practice Questions
Here are some practice questions to help you prepare for the PCAP™ - Certified Associate Python Programmer certification. This certification focuses on Object-Oriented Programming using Python and covers advanced topics such as OOP essentials, module and package essentials, exception handling, advanced string operations, list comprehensions, lambdas, generators, closures, and file processing.
Having a PCAP™ certification will boost your confidence in your programming skills, make you more competitive in the job market, and give you a head start in preparing for and advancing to the professional level.
Below are some sample questions from MyExamCloud's PCAP Practice Tests Study Plan. To access brief explanations for these questions, take the free PCAP (PCAP-31-03) Practice Mock Exam.
Topic:Section 1: Modules and Packages
QUESTION: 1
Code Given:
from math import pi
def sin(x):
print('sin called')
return 0.99999999
sin(pi / 2)
What is the output?
A:Name conflict error will be thrown
B:No output will be displayed
C:sin called
D:None of the above
ANSWER: Choice C
Topic:Section 1: Modules and Packages
QUESTION: 2
Which platform function returns your Python environment operating system name?
A:platform.machine(0, 1)
B:platform.machine(1)
C:platform.platform(0, 1)
D:platform.platform()
E:platform.platform(1)
F:platform.machine()
ANSWER: Choice C,Choice D,Choice E
Topic:Section 1: Modules and Packages
QUESTION: 2
Which command will uninstall packagename?
A:pip un-install packagename
B:pip uninstall packagename
C:pip un_install packagename
D:pip --uninstall packagename
E:pip freeze packagename
F:pip --remove packagename
ANSWER: Choice B
Topic:Section 1: Modules and Packages
QUESTION: 3
How do you call the function get_pizza()
saved as garlicpizza.py
below?
def get_pizza():
print("Garlic Pizza")
A:import garlicpizza; get_pizza()
B:import garlicpizza.get_pizza; get_pizza()
C:import get_pizza from garlicpizza; get_pizza()
D:import garlicpizza; garlicpizza.get_pizza()
E:from garlicpizza import get_pizza; get_pizza()
ANSWER: Choice D,Choice E
Topic:Section 1: Modules and Packages
QUESTION: 4
Which module defines functions for generating pseudorandom numbers?
A:random
B:crandom
C:cmath
D:math
ANSWER: Choice A
Topic:Section 1: Modules and Packages
QUESTION: 5
Code Given:
from math import ceil, floor, trunc
n1 = 1.2
n2 = 2.2
print(ceil(n1), ceil(n2))
print(ceil(-n1), ceil(-n2))
What is the output?
A:1 2
-1 -2
B:-1 -2
2 3
C:Error
D:-2 -3
-1 -2
E:2 3
-1 -2
ANSWER: Choice E
Topic:Section 2: Exceptions
QUESTION: 6
Code Given:
price = float(input("Enter product price: "))
assert price >= 1.0
print(price)
What is the output if price = -1?
A:FloatingPointError
B:-1Exception
C:AssertionError
D:BaseException
ANSWER: Choice C
Topic:Section 2: Exceptions
QUESTION: 7
What will happen when an operation cannot be completed due to a lack of free memory?
A:Python will not handle out of memory
B:Python raises MemoryError
C:Python raises OutOfMemoryError
D:Python terminate the operation
ANSWER: Choice B
Topic:Section 2: Exceptions
QUESTION: 8
Which code snippet raises the exception named MyException
?
A:throws MyException
B:throw MyException
C:raise MyException
D:raises MyException
ANSWER: Choice C
Topic:Section 2: Exceptions
QUESTION: 9
Which exception is raised when the user uses a keyboard shortcut designed to terminate a program's execution?
A:InterruptError
B:KeyboardInterruptException
C:KeyboardInterruptError
D:KeyboardInterrupt
ANSWER: Choice D
Topic:Section 3: Strings
QUESTION: 10
What is the output of the following code?
print("www.myexamcloud.com".replace("com", "org"))
print("Python version 2".replace("2 ", "3"))
print("PCAP Certification".replace('PCAP', 'PCPP1'))
A:www.myexamcloud.org
Python version 3
PCPP1 Certification
B:www.myexamcloud.org
Python version 2
PCAP Certification
C:www.myexamcloud.org
Python version 2
PCPP1 Certification
D:www.myexamcloud.org
Python version 3
PCAP Certification
ANSWER: Choice C
Topic:Section 3: Strings
QUESTION: 11
Code Given:
name = 'MyExamCloud Python Certification Courses are good for self-preparation.';
Which of the following code snippets will print Courses found?
A:if name.find('Courses', name.find('Certification')) == True:
print('Courses found')
B:if name.find('Courses', name.find('Certification')) > -1:
print('Courses found')
C:if name.finds('Courses', name.find('Certification')) > -1:
print('Courses found')
D:if name.find('Courses') > -1:
print('Courses found')
E:if name.find('Courses') == True:
print('Courses found')
ANSWER: Choice B,Choice D
Topic:Section 3: Strings
QUESTION: 12
Code Given:
str = ['My', 'Exam', 'Cloud']
s = '*'.join(str)
print(s)
What is the output?
A:My*Exam*Cloud
B:My Exam Cloud
C:My* Exam* Cloud
D:My *Exam *Cloud
ANSWER: Choice A
Topic:Section 3: Strings
QUESTION: 13
What is the output of the following code?
p_list = [
'PCEP',
'PCAP'
'PCPP1'
]
print(p_list)
A:['PCEP', 'PCAPPCPP1']
B:SyntaxError: invalid syntax
C:['PCEP', 'PCAP', 'PCPP1']
D:['PCEP']
ANSWER: Choice A
Topic:Section 3: Strings
QUESTION: 14
Code Given:
str = '''Python is a good language.
PCAP is a good certification.''';
Which of the following code prints True ?
A:print('Certification' in str)
B:print('PCAP' in str)
C:print('Good' in str)
D:print('Python' in str)
ANSWER: Choice B,Choice D
Topic:Section 3: Strings
QUESTION: 15
Code Given:
print('myexamcloud' > 'MyExamCloud')
print('MyExamCloud Courses' < 'MyExamCloud')
print('MyExamCloud' > '100')
print('200' < '100')
What is the output?
A:True
True
True
False
B:True
False
False
False
C:True
False
True
False
D:True
False
True
True
ANSWER: Choice C
Topic:Section 3: Strings
QUESTION: 16
Code Given:
print("MyExamCloud".islower())
print('myexamcloud'.islower())
print('myexamcloud'.isspace())
print("My Exam Cloud".isspace())
print(' \n '.isspace())
print('myexamcloud'.isupper())
print("MyExamCloud".isupper())
What is the output?
A:True
True
False
False
True
False
False
B:False
True
False
False
True
False
True
C:False
True
False
False
True
False
False
ANSWER: Choice C
Topic:Section 4: Object-Oriented Programming
QUESTION: 17
What is the output of the below code?
class Account:
__counter = 0
def __init__(self, type=1):
self.__type = type
Account.__counter += 1
ac1 = Account()
ac2 = Account(1)
ac3 = Account(2)
print(ac1.counter)
print(ac2.counter)
print(ac3.counter)
A:1
3
3
B:3
3
3
C:2
2
2
D:AttributeError
E:1
2
3
ANSWER: Choice D
Topic:Section 4: Object-Oriented Programming
QUESTION: 18
What is the output of the following code?
class Account:
def acc(self):
print('Account')
class PremiumAccount:
def acc(self):
print('PremiumAccount')
class SuperPremiumAccount(Account, PremiumAccount):
def acc(self):
super().acc()
s = SuperPremiumAccount()
s.acc()
A:Account
B:Account PremiumAccount
C:PremiumAccount
D:No output
E:PremiumAccount Account
ANSWER: Choice A
Topic:Section 4: Object-Oriented Programming
QUESTION: 19
What is the output of the following code?
class Account:
def __init__(self, accn=1):
self.accno = accn
def set_custid(self, id):
self.custid = id
acc1 = Account()
acc2 = Account(100)
acc2.set_custid(1)
acc3 = Account(200)
acc3.phone = '9000000'
print(acc1.__dict__)
print(acc2.__dict__)
print(acc3.__dict__)
A:{'accno': 1}
{'accno': 100, 'custid': 1}
{'accno': 200, 'custid': 1}
B:{'accno': 1}
{'accno': 100, 'custid': 1}
Error, no field named 'phone' in Account class
C:{'accno': 1}
{'accno': 100, 'custid': 1}
{'accno': 200, 'phone': '9000000'}
ANSWER: Choice C
Topic:Section 4: Object-Oriented Programming
QUESTION: 20
Which options will display Student given the following code?
class Student:
def __str__(self): return "Student"
s = Student()
A:print(Student().str())
B:print(s)
C:print(s.__dict__)
D:print(Student.__str__())
E:print(s.__str__())
ANSWER: Choice B,Choice E
Topic:Section 5: Misellaneous
QUESTION: 21
Which exception is raised when any file operations fails?
A:SystemError
B:IOError
C:EnvironmentError
ANSWER: Choice B
Topic:Section 5: Misellaneous
QUESTION: 22
What is the output of the code?
for app in open('app.txt', 'rt'):
print(app, end=")
### Content of app.txt ###
MyExamCloud
My Exam Preparation Mentor
A:MyExamCloud My Exam Preparation Mentor is printed in a single line
B:Nothing is printed
C:MyExamCloud and My Exam Preparation Mentor is printed in separate lines
D:TypeError: 'TextIOWrapper' object is not iterable
E:MyExamCloud is printed in an infinite loop
ANSWER: Choice C
Topic:Section 5: Misellaneous
QUESTION: 23
What is true about mkdir function?
A:It creates a directory in the path passed as its argument.
B:The path can be either relative or absolute.
C:All of the above.
ANSWER: Choice C
Topic:Section 5: Misellaneous
QUESTION: 24
Which code snippet produces 2022/01/01 output?
from datetime import date
d = date(2022, 1, 1)
# code here
print(fd)
A:fd = d.str('%Y/%m/%d')
B:fd = d.strftime('%Y/%m/%d')
C:fd = d.format('%Y/%m/%d')
ANSWER: Choice B
Author | JEE Ganesh | |
Published | 6 months ago | |
Category: | Python Certification | |
HashTags | #Python #PythonCertification |