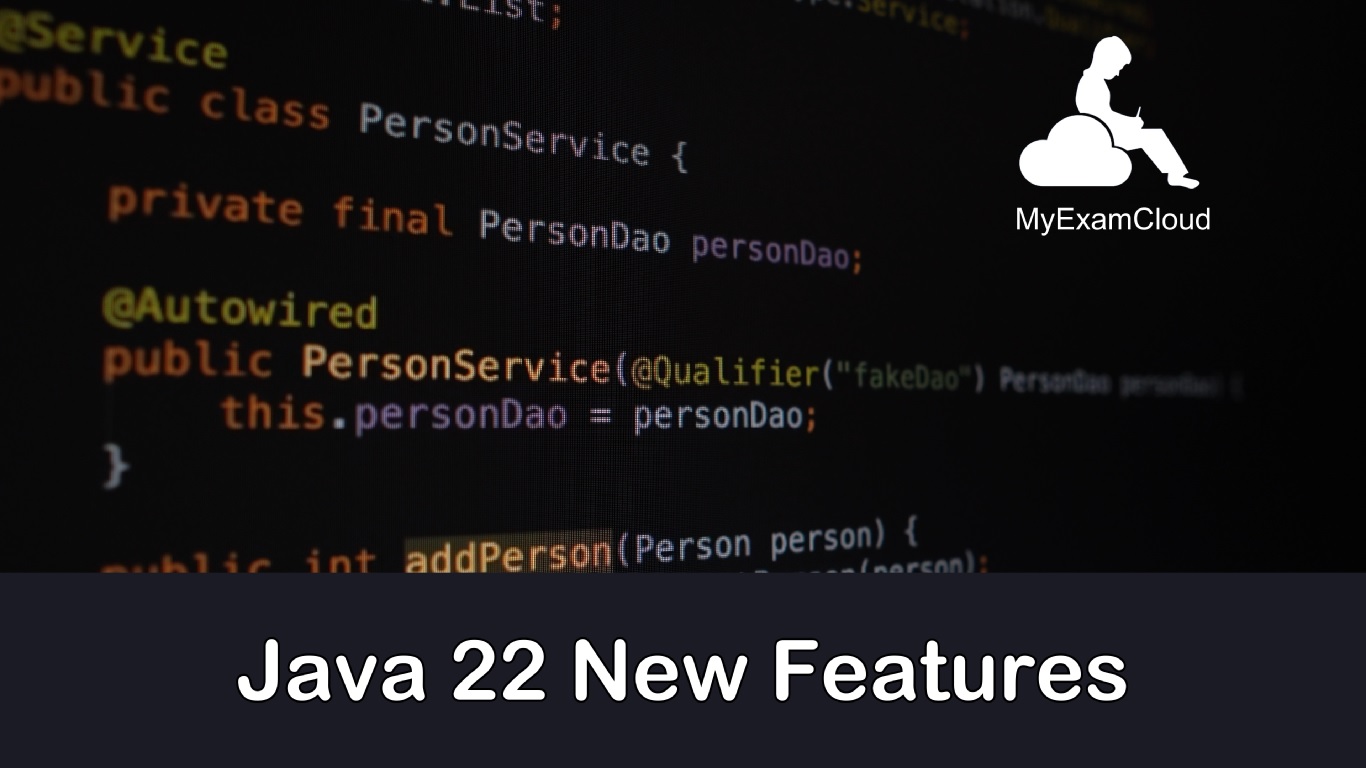
Exploring the Java 22 Feature: Statements Before Super()
Statements before super() is a new Java 22 feature that allows developers to execute statements before calling super() in a constructor of a derived class. This gives more flexibility and simplifies the code as there is no longer a need to create separate static methods or blocks just to check arguments before passing them to the superclass constructor.
Traditionally, in earlier versions of Java, developers were not allowed to execute any statements before calling super() in a constructor. This could be restrictive, especially when there was a need to validate arguments being passed to the superclass constructor. In such cases, developers had to create static methods or blocks to check the values before they could be passed to super(). This added extra code and could be confusing and cumbersome to maintain.
With the new feature, developers can now include validation or other logic directly in the constructor of a derived class, right before calling super(). This makes the code more concise and readable, eliminating the need for separate static methods for validation or any other tasks that need to be executed before calling super().
Let's take a look at an example where this feature can be useful. Suppose we have a Vehicle class with two instance variables, wheels and type, which are initialized through the constructor. In the constructor, we want to ensure that the type of vehicle is always provided. To do this, we can write a simple if-statement before calling super() to verify that the type is not empty.
package statementsBeforeSuper;
public class Vehicle {
private int wheels;
private String type;
// constructor with validation before super()
public Vehicle(int wheels, String type) {
if(type.isEmpty()) {
throw new IllegalArgumentException("Type of vehicle cannot be empty");
}
this.wheels = wheels;
this.type = type;
}
public void displayInfo() {
System.out.println("Vehicle type: " + type + " with " + wheels + " wheels.");
}
}
class Car extends Vehicle {
public Car(int wheels, String type) {
super(wheels, type);
}
}
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car(4, "SUV");
myCar.displayInfo();
}
}
Output:
Vehicle type: SUV with 4 wheels.
👉 In the above example, we have written a validation statement before calling super(), which checks that the type of vehicle is not empty. If it is empty, it throws an exception. This ensures that the type of vehicle is always provided and it avoids creating a car object with an empty type, which could lead to errors later on.
This feature proves to be highly beneficial as it allows for more complex validations or logic to be executed before calling super(). The only restriction is that the statements executed before super() cannot involve any instance variables or methods of the derived class. If this rule is violated, the code will not compile as it tries to access properties of the derived class before the superclass has been properly initialized.
To demonstrate this, let's modify the previous example slightly by moving the if-statement inside the constructor of the Car class and using an instance variable in the if-condition.
Main.java
package statementsBeforeSuper;
class Vehicle {
private int wheels;
private String type;
// constructor with validation
public Vehicle(int wheels, String type) {
this.wheels = wheels;
this.type = type;
}
public void displayInfo() {
System.out.println("Vehicle type: " + type + " with " + wheels + " wheels.");
}
}
class Car extends Vehicle {
private boolean needsRepair = true;
// constructor with validation before super()
public Car(int wheels, String type) {
if(needsRepair) {
throw new IllegalStateException("Cannot create car while it needs repair");
}
super(wheels, type);
}
}
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car(4, "SUV");
myCar.displayInfo();
}
}
Output:
Exception in thread "main" java.lang.IllegalStateException: Cannot create car while it needs repair at statementBeforeSuper.Car.(Main.java:23) at statementBeforeSuper.Main.main(Main.java:34)
As you can see, the code does not compile as it tries to access an instance variable of the derived class before calling super(), resulting in an error.
In conclusion, the introduction of statements before super() in Java 22 is a useful and convenient feature that simplifies and improves the readability of code. By allowing developers to execute statements before calling the superclass constructor, it eliminates the need for extra static methods or blocks for argument validation and other tasks, making the code more concise and efficient. However, it is essential to remember the restriction of not using any instance variables or methods from the derived class before calling super().
Java Certifications Practice Tests - MyExamCloud Study Plans
Python Certifications Practice Tests - MyExamCloud Study Plans
AWS Certification Practice Tests - MyExamCloud Study Plans
Google Cloud Certification Practice Tests - MyExamCloud Study Plans
Aptitude Practice Tests - MyExamCloud Study Plan
MyExamCloud AI Exam Generator
Author | JEE Ganesh | |
Published | 9 months ago | |
Category: | Programming | |
HashTags | #Java #Programming #Software |