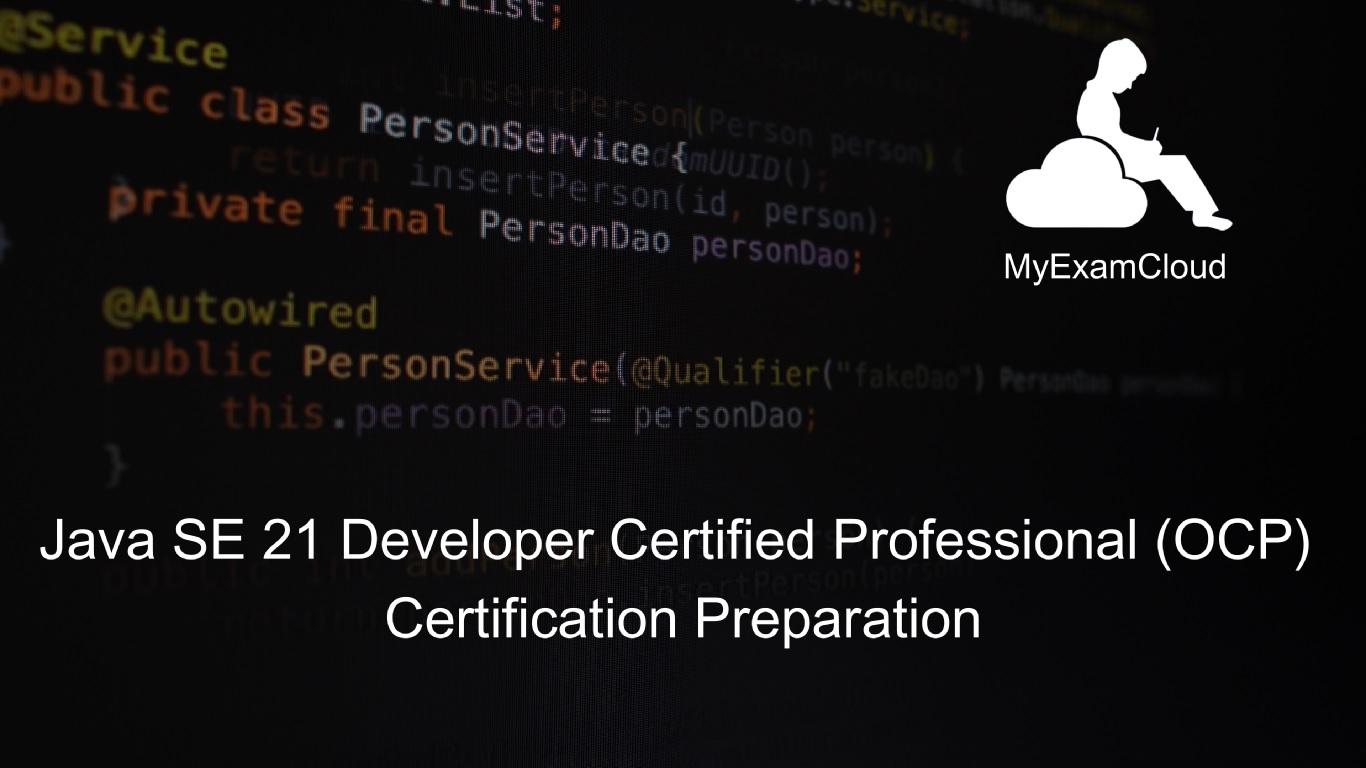
Exploring the New Features in Java SE 21 for Developers and OCP Certification Preparation
Java SE 21 Developer Certified Professional (OCP) Certification is the latest certification offered by Oracle for Java developers. It is a professional-level certification that focuses on advanced features and techniques of Java programming. In this article, we will cover the new features included in Java SE 21, which are also covered in the OCP certification.MyExamCloud 1Z0-830 Practice Tests | Java SE 21 Developer Certified Professional Mock Questions
1. Records
Records are a new feature introduced in Java SE 16 and now finalized in Java SE 21. They are a compact and efficient way to create data classes, where the primary purpose is to hold data. Records are immutable by default, making them an ideal choice for modeling objects with a fixed set of properties such as data transfer objects, domain entities, and data containers.
Records also come with a built-in support for pattern matching, allowing us to easily destructure and match against record instances. This feature is particularly useful for creating more concise and readable code.
Let's take a look at an example:
public record Car(String make, String model, int year) { }
// create an instance of Car
Car car = new Car("Toyota", "Camry", 2021);
// use pattern matching to destructure the record instance and access its properties
if (car instanceof Car(String make, String model, int year)) {
System.out.println(make); // prints "Toyota"
System.out.println(year); // prints 2021
}
2. Pattern Matching for Switch
Pattern matching for switch is another new feature introduced in Java SE 16 and improved in Java SE 21. It allows us to use patterns in switch case labels, making switch statements and expressions more expressive.
By using pattern matching for switch, we can match against complex objects and easily extract and use their properties. This feature also supports matching against null values, making it easier to handle NullPointerException.
Here's an example:
class Account {
double getBalance() {
return 0;
}
}
class SavingsAccount extends Account {
double getSavings() {
return 100;
}
}
// using pattern matching for switch to handle different types of Accounts
public static double getBalance(Account account) {
double result = switch (account) {
case null -> throw new RuntimeException("Account is null");
case SavingsAccount sa -> sa.getSavings();
default -> account.getBalance();
};
return result;
}
3. String Templates
String templates are a new feature introduced in Java SE 21, aimed at improving the way we compose strings in Java. They allow us to combine literal text with template expressions and processors to produce the desired result.
Java provides three built-in template processors: STR, FMT, and RAW. We can use these processors to evaluate template expressions and convert them into strings, making our code more concise and readable.
An example of string templates in action:
String name = "John";
// using string template with STR processor
String greeting = STR."Hello, \{name}"; // evaluates to "Hello, John"
// using string template with FMT processor
String output = FMT."Format as: \{"Hello, %s"}".format(name); // evaluates to "Hello, John"
// using string template with RAW processor
String statement = RAW."Name is: \{$name}"; // evaluates to "Name is: John"
4. Virtual Threads
Virtual threads, also known as Project Loom, are a new feature added in Java SE 21. They are a lightweight alternative to platform threads, aimed at improving scalability and concurrency in Java applications.
Virtual threads have the ability to share OS threads, making them more efficient in handling large numbers of concurrent tasks. They also come with support for Thread Local Variables, making it easier to handle thread-specific data.
An example of using virtual threads in Java:
// create a virtual thread executor
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
IntStream.rangeClosed(1, 10_000).forEach(i -> {
// submit tasks to the executor
executor.submit(() -> System.out.println(i));
});
}
5. Sequenced Collections
Java SE 21 introduces three new interfaces to represent sequenced collections, sequenced sets, and sequenced maps. These interfaces (Sequenced, SequencedSet, SequencedMap) extend the Collection interface and provide a defined encounter order for their elements.
Sequenced Sets and Maps, similar to their counterparts (LinkedHashSet and SortedMap) in the Collections framework, do not allow duplicates and maintain a defined order for their elements. Sequenced Collections, on the other hand, allow duplicates and maintain a specific order for their elements.
An example of creating and using a sequenced collection:
// create a sequenced collection
Sequenced<String> sequence = new SequencedArrayList<>();
// add elements in a specific order
sequence.add("element 1");
sequence.add("element 2");
// access the first and last elements
String first = sequence.first(); // returns "element 1"
String last = sequence.last(); // returns "element 2"
// iterate through all elements in the specified order
for (String element : sequence) {
System.out.println(element);
}
6. Key Encapsulation Mechanism API
The Key Encapsulation Mechanism (KEM) API, introduced in Java SE 21, allows applications to use algorithms for generating secure symmetric keys using asymmetric keys. It provides a more secure way of handling symmetric keys, making it easier to implement secure encryption and decryption in Java applications.
The KEM API supports a variety of algorithms, including NIST KEMs, some custom algorithms provided by vendors, and user-provided algorithms. It also provides a standard way of handling exceptions and errors while using different KEM algorithms.
An example of using the KEM API for generating symmetric keys:
// generate a key encapsulation algorithm using NIST KEM
KeyEncapsulationAlgorithm kemAlgorithm = KEM.newNISTKem();
// generate a key pair for encryption
KeyPair keyPair = kemAlgorithm.generateKeyPair();
// create a shared secret from the key pair
byte[] sharedSecret = kemAlgorithm.generateSecret(keyPair);
// create a symmetric key from the shared secret
SecretKey secretKey = kemAlgorithm.encryptSecret(sharedSecret);
Conclusion
Java SE 21 has brought in some exciting new features that will help developers write more efficient and secure code. These features are also covered in the Java certification, making it essential for Java developers to familiarize themselves with these new additions.
Author | JEE Ganesh | |
Published | 9 months ago | |
Category: | Java Certification | |
HashTags | #Java #Programming #Software #JavaCertification |