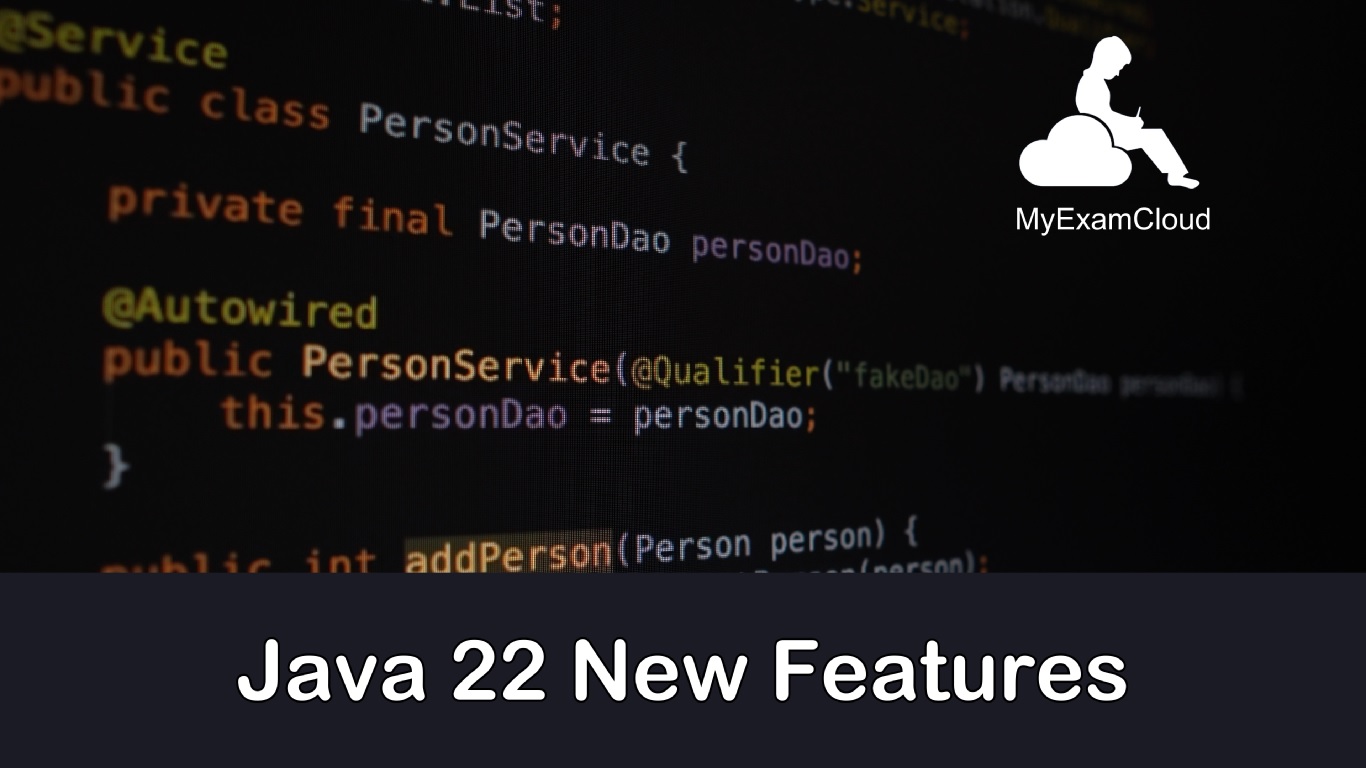
Java 22 Stream Gatherers: A Flexible Approach to Intermediate Operations
Java 22 Stream Gatherers (JEP 461)
Exploring the extensible API for intermediate java.util.stream operations.
Java's Stream API provides a way to process collections of data in a sequence of steps, similar to a factory assembly line. The pipeline starts with a source, passes through intermediate operations, and ends with a terminal operation. Stream Gatherers, introduced in Java 22, offer a generic approach for creating intermediate operations, similar to how the Collectors API provides a generic way to define terminal operations.
Understanding through a Basic Example:
Let's say we have a list of names and we want to find the length of the longest name that starts with the letter "J". We can set up a stream pipeline using Java 22's Stream Gatherers to achieve this:
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List names = Arrays.asList("Julia", "John", "Jessica", "Alexis", "Jayden");
int longest = names.stream() // Create a stream from the list
.filter(n -> n.startsWith("J")) // Intermediate operation: keep only names starting with "J"
.gather(Gatherers.maxBy(String::length)) // Intermediate operation: find the longest name
.map(String::length) // Transform the result to the length of the name
.get(); // Terminal operation: get the final result
System.out.println("Length of the longest name starting with \"J\": " + longest);
}
}
Purpose and Functionality:
The Gatherer interface is heavily influenced by the design of the Collector interface in the Collectors API. Gatherers allow for the transformation and processing of stream elements in customizable ways, similar to how Collectors allow for terminal operations.
Gatherer Interface:
Interface Gatherer
The Gatherer interface takes three type parameters:
S → the input type (the type of element that it consumes)
A → the state type of the gatherer operation (often hidden as an implementation detail)
R → what type of element this gatherer produces
Methods in the Gatherer Interface:
Initializer Function (optional): This function sets up a private "state" object that remembers information needed for processing elements in the stream.
Integrator Function: This is the main function where each element of the stream is processed. It uses the state object and can add new elements to the output or even stop the stream early.
Combiner Function (optional): Used to merge the results from parallel processing.
Finisher Function (optional): Wraps up the processing after all elements have been processed.
Built-in Gatherers:
Java 22's Stream Gatherers class provides several built-in gatherers:
fold: Combines all the input elements into a single result and returns this result once all input is processed.
mapConcurrent: Processes each input element individually, making it efficient for parallel processing of large datasets.
scan: Allows for continuous updating of results as each element is processed.
windowFixed: Groups elements into fixed-size lists, useful for batch processing.
windowSliding: Similar to windowFixed, but with overlapping groups, useful for analyzing data over a moving range.
Understanding through an Example:
Problem Statement:
We have a list of movies, and we want to organize them into groups of two. We only want the first two groups.
With Stream Gatherers:
package streamGatherers;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Gatherers;
import java.util.stream.Stream;
public class Main {
public static List> findGroupsOfTwoWithGatherer(List input, int groupSize, int numberOfGroups) {
return Stream.iterate(0, i -> i + 1)
.map(index -> input.get(index))
.limit(input.size())
.gather(Gatherers.windowFixed(groupSize))
.limit(numberOfGroups)
.collect(Collectors.toList());
}
public static void main(String[] args) {
List movies = List.of(
"The Shawshank Redemption",
"The Godfather",
"The Godfather: Part II",
"The Dark Knight",
"12 Angry Men",
"Schindler's List",
"The Lord of the Rings: The Return of the King"
);
List> grouped = findGroupsOfTwoWithGatherer(movies, 2, 2);
System.out.println(grouped);
}
}
Output:
[[The Shawshank Redemption, The Godfather], [The Godfather: Part II, The Dark Knight]]
Conclusion: Java 22's Stream Gatherers offer a flexible and efficient way to process collections of data in intermediate operations of a stream pipeline. The Gatherer interface and its built-in gatherers provide a versatile tool for a variety of complex transformations and analysis of data.
Java Certifications Practice Tests - MyExamCloud Study Plans
Python Certifications Practice Tests - MyExamCloud Study Plans
AWS Certification Practice Tests - MyExamCloud Study Plans
Google Cloud Certification Practice Tests - MyExamCloud Study Plans
Aptitude Practice Tests - MyExamCloud Study Plan
MyExamCloud AI Exam Generator
Author | JEE Ganesh | |
Published | 9 months ago | |
Category: | Programming | |
HashTags | #Java #Programming #Software |